Pointers in Programming: Essential Concepts and Memory Management - Part 1
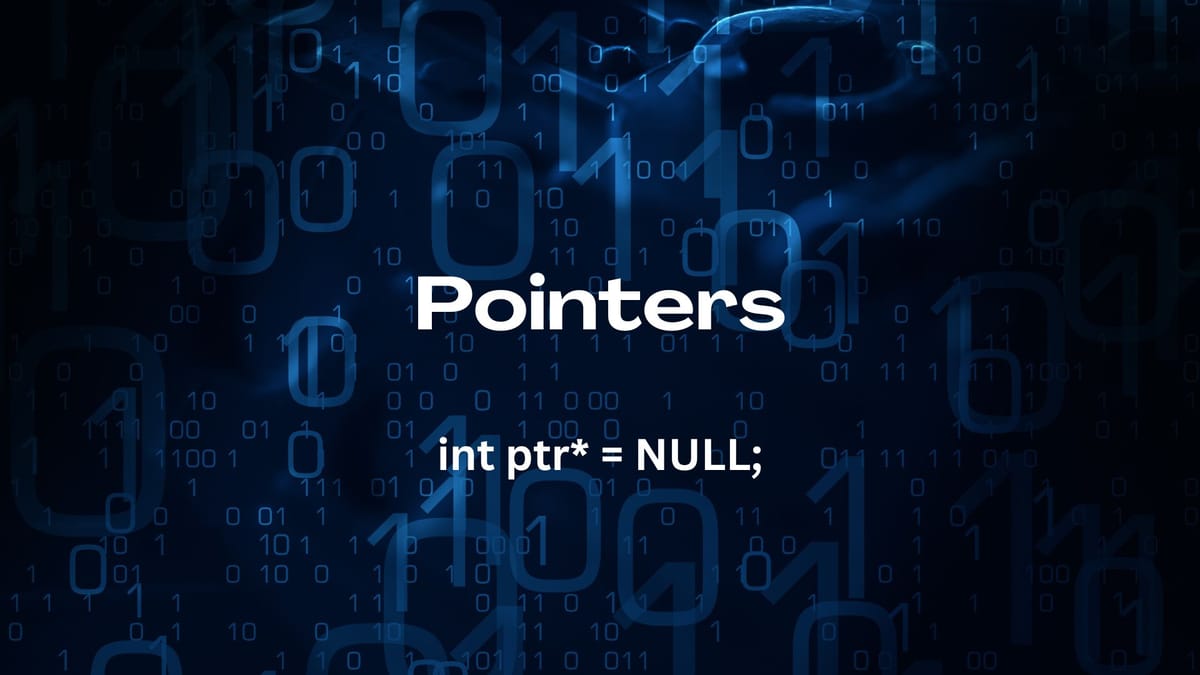
If you've delved into the world of software engineering, you've likely encountered the term "pointers." This concept is closely tied to what some programming languages refer to as "memory safety," a term often associated with languages like Rust or those with garbage collectors like Go, JavaScript, and others.
But why do pointers have such a bad reputation in some circles? Despite being a fundamental concept since the early days of software engineering, even so-called memory-safe languages use pointers. The difference lies in how these languages manage the lifecycle of pointers and the memory they reference, ensuring that memory is cleaned up once a pointer goes out of scope.
What Are Pointers?
In simple terms, a pointer is a variable that holds the memory address of another variable. While the implementation details may vary across platforms or programming languages, the underlying concept remains the same: pointers are a way to access and manipulate data in the heap memory.
Why Do You Need Pointers?
Memory in computers into different categories, called segments. Here is an excerpt from the learncpp site:
- The code segment (also called a text segment), where the compiled programme sits in memory. The code segment is typically read-only.
- The bss segment (also called the uninitialised data segment), where zero-initialized global and static variables are stored.
- The data segment (also called the initialized data segment), where initialised global and static variables are stored.
- The heap, where dynamically allocated variables are allocated from.
- The call stack, where function parameters, local variables, and other function-related information are stored.
For now, lets focus on the last two, the stack and heap.
- Stack Memory: Think of stack memory as a fixed-size portion allocated to each block for local variable storage. When you declare a variable, the data is "pushed" onto the top of the stack. Once the function returns or the variable goes out of scope, memory is "popped" from the stack. This limited size is usually sufficient for most small, short-lived data, but can be restrictive for larger or more complex data structures.
- Heap Memory: Heap memory, on the other hand, is more flexible but also more complex to manage. It's like a bank reserve where you can request memory of any size at runtime. When you allocate memory on the heap, you're given back a memory address, which is stored in a variable on the stack. This variable is what we call a pointer, as it points to a location on the heap.
The challenge with heap memory arises when the pointer goes out of scope. While the stack memory used by the pointer is automatically cleaned up, the memory on the heap that the pointer referenced is not automatically freed. This can lead to a situation known as a memory leak, where allocated memory is never released, leading to inefficient memory usage and potentially exhausting the available memory over time.
The Dangers of mishandling Pointers
Pointers, while powerful, come with significant risks if misused:
- Security Vulnerabilities: Improper pointer handling can lead to serious security issues. For instance, if a pointer is used after the memory it references has been freed (a "dangling pointer"), this can lead to undefined behavior and vulnerabilities that malicious actors can exploit.
- Memory Corruption: Incorrectly managing pointers can cause memory corruption, where the data in memory becomes inconsistent or invalid. This can lead to unpredictable behavior and application crashes.
- Application Crashing: Misuse of pointers can easily lead to application crashes, particularly if a programme tries to access memory it shouldn't or if it fails to handle memory allocation errors properly.
How Do Garbage-Collected Languages Handle Pointers?
In languages like C and C++, pointer management is the programmer's responsibility. This means ensuring that any memory allocated is eventually freed, which can be error-prone and tedious.
Garbage-collected languages, such as Java, Go, and JavaScript, automate this process. They use a mechanism to track which pieces of memory are still in use and which can be safely reclaimed. The specifics vary between languages:
- Pause-and-Sweep: Some languages implement a "stop-the-world" garbage collection approach, where program execution pauses while the system identifies and reclaims unused memory. While effective, this can lead to noticeable pauses in application performance.
- Concurrent Garbage Collection: To minimize pauses, some languages use concurrent garbage collection, where the system reclaims memory in the background while the program continues to run. This reduces the impact on performance, but the trade-offs depend on the language's specific implementation.
In essence, while pointers remain a crucial part of programming, understanding and properly managing them is essential to avoid the pitfalls that can lead to insecure, unstable, or inefficient applications.